Question & Examples
Write a function called sumZero which accepts a sorted array of integers. The function should find the first pair where the sum is 0. Return an array that includes both values that sum to zero or undefined if a pair doesn't exist.
// Examples
sumZero([-3, -2, -1, 0, 1, 2, 3]) // [-3, 3]
sumZero([-2, 0, 1, 3]) // undefined
sumZero([1, 2, 3]) // undefined
My Solution
function sumZero(arr) {
if(arr.length === 0) {
return undefined
}
let pointer1
let pointer2
for(let i = 0; i < arr.length; i++) {
pointer1 = i
for(let j = i + 1; j < arr.length; j++) {
pointer2 = j
if(arr[pointer1] + arr[pointer2] === 0) {
return [arr[pointer1], arr[pointer2]]
}
}
}
return undefined
}
My solution involves using pointers and looping over a loop. It's pretty straightforward, but not the optimal solution.
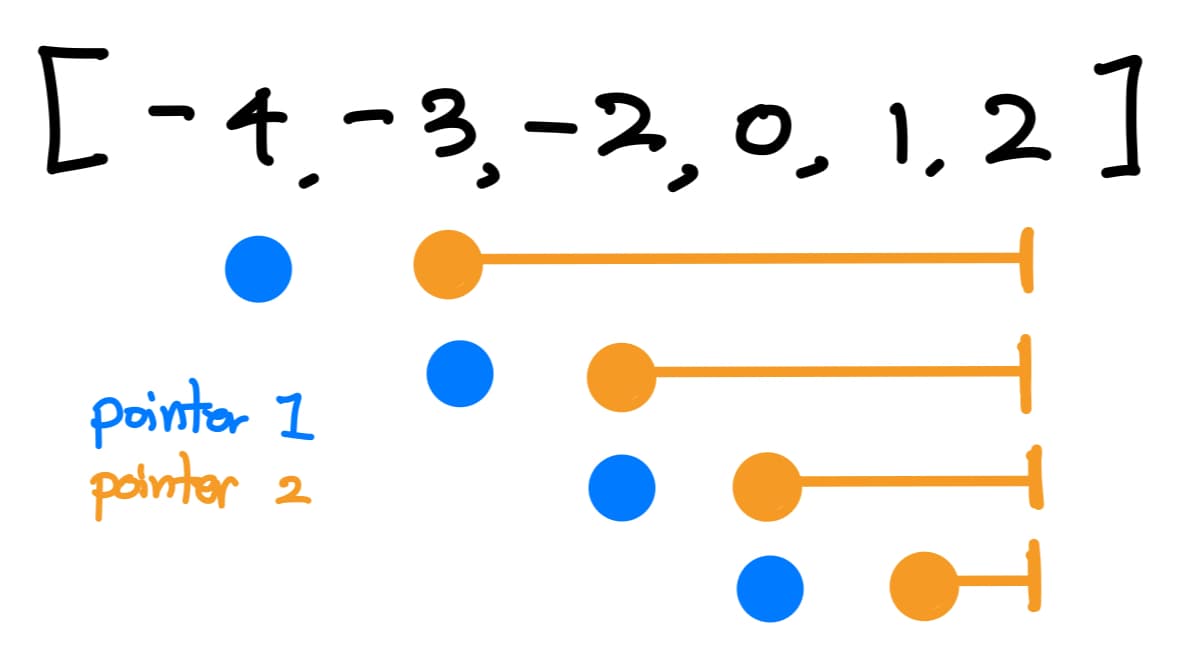
pointer1
starts at the very beginning of the array and pointer2
starts at the index of pointer1 + 1. The second pointer will loop over the entire array and if we don't find a match, pointer1
is assigned with the index of 1 and pointer2
with index of 2 and so forth...
A Better Solution
The length of our array and the time complexity are positively correlated, which is not good. Time Complexity is O(n^2) which is not optimal. A better solution I found out was this:
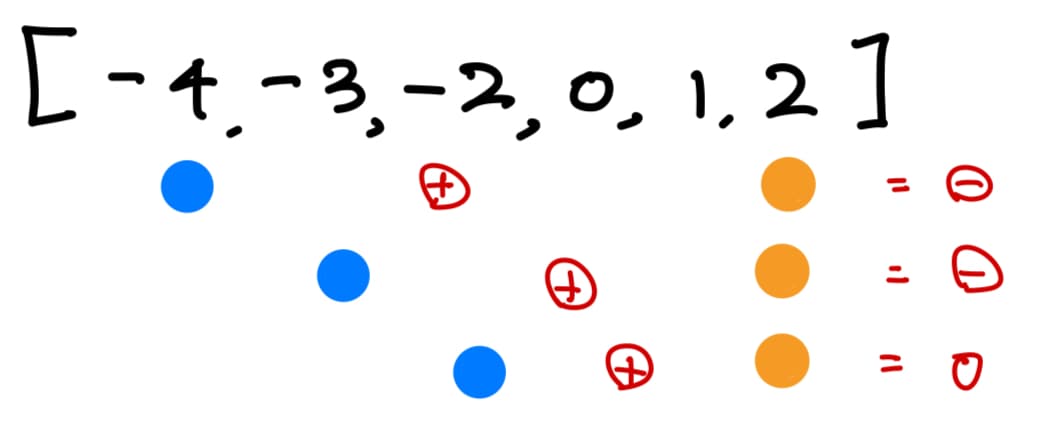
We could start pointer1
as it is. However, we would start pointer2
at the end of the array. We would then take the values of each pointers and add them. If the added value is a...
- negative value, we will move
pointer1
+ 1 - positive value, we will move
pointer2
- 1
In the example above, we start with pointer1
and pointer2
in their respective positions.
First, we add them and get a negative value. We will then move pointer1
left by 1. We add the two values again and compare. Add again and compare and so on... We do this until we get the value of 0. If this doesn't happen, we return undefined.
function sumZero(arr) {
if(arr.length === 0) {
return undefined
}
let pointer1 = 0;
let pointer2 = arr.length - 1
// constantly check to see if pointer2 surpasses pointer1
while (pointer1 < pointer2) {
let sum = arr[pointer1] + arr[pointer2]
if(sum === 0) {
return [arr[pointer1], arr[pointer2]]
} else if(sum > 0) {
pointer2--
} else {
pointer1++
}
}
return undefined
}
Time complexity is O(n)